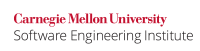
Compound operations on shared variables (consisting of more than one discrete operation) must be performed atomically. Errors can arise from compound operations that need to be perceived atomically but are not [[JLS 05]].
Compound assignment expressions include operators *=, /=, %=, +=, -=, <<=, >>=, >>>=, ^=, or |=
. The postfix and prefix increment (++
) and decrement (--
) operations can also be treated as compound expressions.
For atomicity of a grouping of calls to independently atomic methods of the existing Java API, see CON07-J. Do not assume that a grouping of calls to independently atomic methods is atomic.
The Java Language Specification also permits reads and writes of 64-bit values to be non-atomic though this is not an issue with most modern JVMs (see CON25-J. Ensure atomicity when reading and writing 64-bit values).
Noncompliant Code Example (bitwise compound operation)
This noncompliant code example declares a shared boolean
variable flag
and uses an optimization in the toggle()
method to negate the current value of the flag.
class Foo { private boolean flag = true; public void toggle() { // unsafe flag ^= true; // same as flag = !flag; } public boolean getFlag() { // unsafe return flag; } }
However, this code is not thread-safe. Multiple threads may not observe the latest state of the flag
because ^=
constitutes a non-atomic operation.
For example, consider two threads that call toggle()
. Theoretically the effect of toggling flag
twice should restore it to its original value. But the following scenario could occur, leaving flag
in the wrong state.
Time |
flags= |
Thread |
Action |
---|---|---|---|
1 |
true |
t1 |
reads the current value of |
2 |
true |
t2 |
reads the current value of |
3 |
true |
t1 |
toggles the temporary variable to false |
4 |
true |
t2 |
toggles the temporary variable to false |
5 |
false |
t1 |
writes the temporary variable value to |
6 |
false |
t2 |
writes the temporary variable value to |
As a result, the effect of the call by t1 is not reflected in flag
; the program behaves as if the call was never made.
Noncompliant Code Example (volatile
variable)
This noncompliant code example derives from the preceding one but declares the flag
as volatile
.
class Foo { private volatile boolean flag = true; public void toggle() { // unsafe flag ^= true; } public boolean getFlag() { // safe return flag; } }
It is still insecure for multithreaded use because volatile
does not guarantee the visibility of updates to the shared variable flag
when a compound operation is performed.
Compliant Solution (synchronization)
This compliant solution guards reads and writes to the flag
field with a lock on the instance, i.e., this
. This is accomplished by declaring both methods to be synchronized
. This solution, via locking, ensures that changes are visible to all the program's threads.
class Foo { private boolean flag = true; public synchronized void toggle() { flag ^= true; // same as flag = !flag; } public synchronized boolean getFlag() { return flag; } }
Compliant Solution (java.util.concurrent.atomic.AtomicBoolean
)
This compliant solution uses the java.util.concurrent.atomic.AtomicBoolean
type to declare the flag
.
class Foo { private AtomicBoolean flag = new AtomicBoolean(true); public void toggle() { boolean temp; do { temp = flag.get(); } while(!flag.compareAndSet(temp, !temp)); } public AtomicBoolean getFlag() { return flag; } }
It ensures that updates to the variable are carried out by using the compareAndSet()
method of the class AtomicBoolean
. All updates are made visible to other threads.
Noncompliant Code Example (addition)
In this noncompliant code example, the two fields a
and b
may be set by multiple threads, using the setValues()
method.
class Adder { private int a; private int b; public int getSum() { return a + b; } public void setValues(int a, int b) { this.a = a; this.b = b; } }
The getSum()
method may return a different sum every time it is invoked from different threads. For instance, if a
and b
currently have the value 0, and one thread calls getSum()
while another calls setValues(1, 1)
, then getSum()
might return 0, 1, or 2. Of these, the value 1 is unacceptable; it is returned when the first thread reads a
and b
, after the second thread has set the value of a
but before it has set the value of b
.
Note that declaring the variables as volatile
does not resolve the issue. Also, this code does not prevent integer overflow. See INT00-J. Perform explicit range checking to ensure integer operations do not overflow for more information.
Noncompliant Code Example (overflow check, atomic integer fields)
The issues described in the previous noncompliant code example can also arise when the fields a
and b
of type int
are replaced with atomic integers.
class Adder { private final AtomicInteger a = new AtomicInteger(); private final AtomicInteger b = new AtomicInteger(); public int getSum() throws ArithmeticException { // Check for integer overflow if( b.get() > 0 ? a.get() > Integer.MAX_VALUE - b.get() : a.get() < Integer.MIN_VALUE - b.get() ) { throw new ArithmeticException("Not in range"); } return a.get() + b.get(); // or, return a.getAndAdd(b.get()); } public void setValues(int a, int b) { this.a.set(a); this.b.set(b); } }
For example, when a thread is executing setValues()
another may invoke getSum()
and retrieve an incorrect result. Furthermore, in the absence of synchronization, there are data races in the check for integer overflow. For instance, a thread can call setValues()
after a second thread has read a
, but before it has read b
in order to add them together; in which case, the second thread will get an improper addition. Even worse, a thread can call setValues()
after a second thread has verified that overflow will not occur, but before the second thread reads the values to add. This would cause the second thread to add two values that have not been checked for overflow, and overflow when adding them.
Note that even though a check for integer overflow is installed, there is a time-of-check-time-of-use (TOCTOU) condition between the overflow check and the addition operation.
Compliant Solution (addition, synchronized)
This compliant solution synchronizes the setValues()
and getSum()
methods so that the entire operation is atomic.
class Adder { private int a; private int b; public synchronized int getSum() throws ArithmeticException { // Check for integer overflow if( b > 0 ? a > Integer.MAX_VALUE - b : a < Integer.MIN_VALUE - b ) { throw new ArithmeticException("Not in range"); } return a + b; } public synchronized void setValues(int a, int b) { this.a = a; this.b = b; } }
Unlike the noncompliant code example, if a
and b
currently have the value 0, and one thread calls getSum()
while another calls setValues(1, 1)
, getSum()
may return return 0, or 2, depending on which thread obtains the intrinsic lock first. The locking guarantees that getSum()
will never return the unacceptable value 1.
This compliant solution also ensures that there is no TOCTOU condition between checking for overflow and adding the fields.
Risk Assessment
If operations on shared variables are not atomic, unexpected results may be produced. For example, there can be inadvertent information disclosure as one user may be able to receive information about other users.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON01- J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
Dynamic analysis tools with a Java concurrency focus, such as SureLogic Flashlight and Coverity Dynamic Analysis will uncover the race conditions shown in the noncompliant code examples above. To accomplish this, however, these tools would have to observe the noncompliant code being called by two or more threads. Such as in an integration or stress test environment. These tools use a dynamic lockset analysis to detect race conditions. This analysis intersects the set of locks that are observed to be held when each piece of shared state in the program is accessed. If the lockset for a piece of shared state is empty then a race condition may have been observed and the tool reports this to the use.
Heurisitics-based static analysis tools, such as FindBugs and PMD, do not detect problems with the noncompliant solutions shown above without some "hint" that the program code is intended to be thread-safe. For example, consider the compliant code below where the use of a synchronized
method is a hint to the analysis tool that the class is intended to be used concurrently.
public class Foo { private boolean flag = true; public synchronized boolean toggleAndGet() { flag ^= true; // same as flag = !flag; return flag; } }
FindBugs and PMD will not report a warning about this implementation as they do not note any problems.
SureLogic JSure, an analysis-based verification tool, will complain that the lock is unknown to the tool and ask the user to annotate what state the lock protects, i.e., the tool wants to know the locking policy that the programmer intends for this class. To express this intent, the programmer adds two annotations:
@RegionLock("FlagLock is this protects flag") @Promise("@Unique(return) for new()") public class Foo { private boolean flag = true; public synchronized boolean toggleAndGet() { flag ^= true; // same as flag = !flag; return flag; } }
The @RegionLock annotation creates a locking policy, named FlagLock
, that specifies that reads and writes to the field flag
are to be guarded by a lock on the receiver, i.e., this
. The second annotation, @Promise is used to place an annotation on the default constructor generated by the compiler. The @Unique("return") annotation promises that the receiver is not aliased during object construction, i.e., that a race condition cannot occur during construction. (CON14-J. Do not let the "this" reference escape during object construction provides further details.) If the constructor was explicit in the code then the annotations would be:
@RegionLock("FlagLock is this protects flag") public class Foo { private boolean flag; @Unique("return") public Foo() { flag = true; } public synchronized boolean toggleAndGet() { flag ^= true; // same as flag = !flag; return flag; } }
The JSure verification tool provides a strong assurance that the annotated model holds for all possible executions of the program. If the below noncompliant code is later added to the class,
public boolean getValue() { return flag; }
then JSure will report the violation of the locking policy to the user.
If the noncompliant getValue()
method shown above is defined in the code for Foo
, then FindBugs can also report a problem, again if the locking model is annotated. However, it uses a different annotation than JSure.
public class Foo { @GuardedBy("this") private boolean flag = true; public synchronized boolean toggleAndGet() { flag ^= true; // same as flag = !flag; return flag; } public boolean getValue() { return flag; } }
With the @GuardedBy annotation in place, and only with this annotation in place, FindBugs reports that the field is not guarded against concurrent access in the getValue()
method.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class AtomicInteger
[[JLS 05]] Chapter 17, Threads and Locks, section 17.4.5 Happens-before Order, section 17.4.3 Programs and Program Order, section 17.4.8 Executions and Causality Requirements
[[Tutorials 08]] Java Concurrency Tutorial
[[Lea 00]] Sections, 2.2.7 The Java Memory Model, 2.2.5 Deadlock, 2.1.1.1 Objects and locks
[[Bloch 08]] Item 66: Synchronize access to shared mutable data
[[Daconta 03]] Item 31: Instance Variables in Servlets
[[JavaThreads 04]] Section 5.2 Atomic Variables
[[Goetz 06]] 2.3. "Locking"
[[MITRE 09]] CWE ID 667 "Insufficient Locking", CWE ID 413
"Insufficient Resource Locking", CWE ID 366
"Race Condition within a Thread", CWE ID 567
"Unsynchronized Access to Shared Data"
11. Concurrency (CON) 11. Concurrency (CON) CON02-J. Always synchronize on the appropriate object