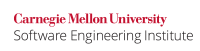
Narrower primitive types can be cast to wider types without any effect on the magnitude of numeric values. However, when the expressions are not strictfp (FLP03-J. Use the strictfp modifier for floating point calculation consistency), conversions from float
to double
may lose information about the overall magnitude of the converted value (see JLS Section 5.1.2, Widening Primitive Conversion).
Conversion from int
or long
to float
, or long
to double
can lead to loss of precision (loss of least significant bits). No runtime exception occurs despite the loss.
Also, see EXP08-J. Be aware of integer promotions in binary operators.
Noncompliant Code Example
In this noncompliant example, an int
is converted to float
. Because a floating point
number cannot be precise to 9 digits, the result of subtracting the original from this value is non-zero.
class WideSample { public static void main(String[] args) { int big = 1234567890; float approx = big; System.out.println(big - (int)approx); // this is expected to be zero but it prints -46 } }
Compliant Solution
The significand part of a floating point
number can hold at most 23 bit values. Anything above this threshold is discarded due to precision loss, as is demonstrated in this compliant solution.
class WideSample { public static void main(String[] args) { int big = 1234567890; float approx = big; if(Integer.highestOneBit(big) <= Math.pow(2, 23)) { //the significand can store at most 23 bits System.out.println(big - (int)approx); //always prints zero now } else { //handle error throw new ArithmeticException("Insufficient precision"); } } }
Risk Assessment
Casting numeric types to wider floating-point types may lose information.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT33- J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Secure Coding Standard as FLP36-C. Beware of precision loss when converting integral types to floating point.
This rule appears in the C++ Secure Coding Standard as FLP36-CPP. Beware of precision loss when converting integral types to floating point.
References
[[JLS 05]] Section 5.1.2, Widening Primitive Conversion
INT31-J. Do not rely on the write() method to output integers outside the range 0 to 255 05. Integers (INT) INT34-J. Perform explicit range checking to ensure integer operations do not overflow