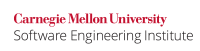
Variables and functions should be declared in the minimum scope from which all references to the identifier are still possible.
When a larger scope than necessary is used, code becomes less readable, harder to maintain, and more likely to reference unintended variables (see DCL01-C. Do not reuse variable names in subscopes).
Noncompliant Code Example
In this noncompliant code example, the function counter()
increments the global variable count
and then returns immediately if this variable exceeds a maximum value:
unsigned int count = 0; void counter() { if (count++ > MAX_COUNT) return; /* ... */ }
Assuming that the variable count
is only accessed from this function, this example is noncompliant because it does not define count
within the minimum possible scope.
Compliant Solution
In this compliant solution, the variable count
is declared within the scope of the counter()
function as a static variable. The static modifier, when applied to a local variable (one inside of a function), modifies the lifetime (duration) of the variable so that it persists for as long as the program does and does not disappear between invocations of the function.
void counter() { static unsigned int count = 0; if (count++ > MAX_COUNT) return; /* ... */ }
The keyword static
also prevents reinitialization of the variable.
Noncompliant Code Example
The counter variable i
is declared outside of the for
loop, which goes against this recommendation because it is not declared in the block in which it is used. If this code were reused with another index variable j
, but there was a previously declared variable i
, the loop could iterate over the wrong variable.
size_t i = 0; for (i=0; i < 10; i++){ /* Perform operations */ }
Compliant Solution
Complying with this recommendation requires that you declare variables where they are used, which improves readability and reusability. In this example, you would declare the loop's index variable i
within the initialization of the for
loop. This requirement was recently relaxed in the C Standard.
for (size_t i=0; i < 10; i++) { /* Perform operations */ }
Noncompliant Code Example (Function Declaration)
In this noncompliant code example, the function f()
is called only from within the function g()
, which is defined in the same compilation unit. By default, function declarations are extern, meaning that these functions are placed in the global symbol table and are available from other compilation units.
int f(int i) { /* Function definition */ } int g(int i) { int j = f(i); /* ... */ }
Compliant Solution
In this compliant solution, the function f()
is declared with internal linkage. This practice limits the scope of the function declaration to the current compilation unit and prevents the function from being included in the external symbol table. It also limits cluttering in the global name space and prevents the function from being accidentally or intentionally invoked from another compilation unit. See DCL15-C. Declare file-scope objects or functions that do not need external linkage as static for more information.
static int f(int i) { /* Function definition */ } int g(int i) { int j = f(i); /* ... */ }
Risk Assessment
Failure to minimize scope could result in less reliable, readable, and reusable code.
Recommendation | Severity | Likelihood | Detectable | Repairable | Priority | Level |
---|---|---|---|---|---|---|
DCL19-C | Low | Unlikely | Yes | Yes | P3 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Astrée | 24.04 | local-object-scope global-object-scope | Partially checked |
Axivion Bauhaus Suite | 7.2.0 | CertC-DCL19 | |
CodeSonar | 9.0p0 | LANG.STRUCT.SCOPE.FILE | Scope could be file static |
1.2 | CC2.DCL19 | Fully implemented | |
Helix QAC | 2024.4 | C1504, C1505, C1531, C1532, C3210, C3218 | |
Klocwork | 2024.4 | MISRA.VAR.MIN.VIS | |
LDRA tool suite | 9.7.1 | 25 D, 61 D, 40 S | Fully implemented |
Parasoft C/C++test | 2024.2 | CERT_C-DCL19-a | Declare variables as locally as possible |
PC-lint Plus | 1.4 | 765, 9003 | Partially supported |
Polyspace Bug Finder | R2024b | Checks for:
Rec. partially covered. | |
PVS-Studio | 7.37 | V821 | |
RuleChecker | 24.04 | local-object-scope global-object-scope | Partially checked |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
SEI CERT C++ Coding Standard | VOID DCL07-CPP. Minimize the scope of variables and methods |
MISRA C:2012 | Rule 8.9 (advisory) |
7 Comments
Jim Gimpel
Just for clarification, can you provide an example of this recommendation applied to a function declaration?
David Svoboda
I suspect the answer is that a function declaration
should not provide names for its parameters.
Even better, it can provide them in comments.
Robert Seacord
I recall seeing this before, but I don't remember the justification. It is certainly common practice to name the parameters, see http://stackoverflow.com/questions/8174886/put-name-of-parameters-in-c-function-prototypes
David Svoboda
Agreed. While I've seen advice not to name the parms (or to name them in comments), I've never seen this used with much force (eg its very weak). On that StackOverflow page the best reason I can see to name the parms (as opposed to naming them in comments) is that they can be easily harvested by an IDE.
Robert Seacord
I think it probably was just meant to suggest that functions that were only called from within the compilation unit should be declared as static. I've added an example.
Joseph C. Sible
We should add an example of a loop counter being legitimately examined after the loop (such as if it contains a
break
), like this:David Svoboda
Looks good...go ahead & add this compliant example.