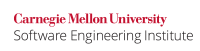
Starting and using background threads during class initialization can result in class initialization cycles and deadlock. For example, the main thread responsible for performing class initialization can block waiting for the background thread, which in turn will wait for the main thread to finish class initialization. This issue can arise, for example, when a database connection is established in a background thread during class initialization [Bloch 2005b]. Consequently, programs must ensure that class initialization is complete before starting any threads.
Noncompliant Code Example (Background Thread)
In this noncompliant code example, the static initializer starts a background thread as part of class initialization. The background thread attempts to initialize a database connection but should wait until all members of the ConnectionFactory
class, including dbConnection
, are initialized.
public final class ConnectionFactory { private static Connection dbConnection; // Other fields ... static { Thread dbInitializerThread = new Thread(new Runnable() { @Override public void run() { // Initialize the database connection try { dbConnection = DriverManager.getConnection("connection string"); } catch (SQLException e) { dbConnection = null; } } }); // Other initialization, for example, start other threads dbInitializerThread.start(); try { dbInitializerThread.join(); } catch (InterruptedException ie) { throw new AssertionError(ie); } } public static Connection getConnection() { if (dbConnection == null) { throw new IllegalStateException("Error initializing connection"); } return dbConnection; } public static void main(String[] args) { // ... Connection connection = getConnection(); } }
Statically initialized fields are guaranteed to be fully constructed before they are made visible to other threads (see TSM03-J. Do not publish partially initialized objects for more information). Consequently, the background thread must wait for the main (or foreground) thread to finish initialization before it can proceed. However, the ConnectionFactory
class's main thread invokes the join()
method, which waits for the background thread to finish. This interdependency causes a class initialization cycle that results in a deadlock situation [Bloch 2005b].
Similarly, it is inappropriate to start threads from constructors (see TSM01-J. Do not let the this reference escape during object construction for more information). Creating timers that perform recurring tasks and starting those timers from within code responsible for initialization also introduces liveness issues.
Compliant Solution (Static Initializer, No Background Threads)
This compliant solution initializes all fields on the main thread rather than spawning background threads from the static initializer.
public final class ConnectionFactory { private static Connection dbConnection; // Other fields ... static { // Initialize a database connection try { dbConnection = DriverManager.getConnection("connection string"); } catch (SQLException e) { dbConnection = null; } // Other initialization (do not start any threads) } // ... }
Compliant Solution (ThreadLocal
)
This compliant solution initializes the database connection from a ThreadLocal
object so that each thread can obtain its own unique instance of the connection.
public final class ConnectionFactory { private static final ThreadLocal<Connection> connectionHolder = new ThreadLocal<Connection>() { @Override public Connection initialValue() { try { Connection dbConnection = DriverManager.getConnection("connection string"); return dbConnection; } catch (SQLException e) { return null; } } }; // Other fields ... static { // Other initialization (do not start any threads) } public static Connection getConnection() { Connection connection = connectionHolder.get(); if (connection == null) { throw new IllegalStateException("Error initializing connection"); } return connection; } public static void main(String[] args) { // ... Connection connection = getConnection(); } }
The static initializer can be used to initialize any shared class field. Alternatively, the fields can be initialized from the initialValue()
method.
Exceptions
TSM02-J-EX0: Programs are permitted to start a background thread (or threads) during class initialization, provided the thread cannot access any fields. For example, the following ObjectPreserver
class (based on [Grand 2002]) provides a mechanism for storing object references, which prevents an object from being garbage-collected even when the object is never again dereferenced.
public final class ObjectPreserver implements Runnable { private static final ObjectPreserver lifeLine = new ObjectPreserver(); private ObjectPreserver() { Thread thread = new Thread(this); thread.setDaemon(true); thread.start(); // Keep this object alive } // Neither this class nor HashMap will be garbage-collected. // References from HashMap to other objects // will also exhibit this property private static final ConcurrentHashMap<Integer,Object> protectedMap = new ConcurrentHashMap<Integer,Object>(); public synchronized void run() { try { wait(); } catch (InterruptedException e) { Thread.currentThread().interrupt(); // Reset interrupted status } } // Objects passed to this method will be preserved until // the unpreserveObject() method is called public static void preserveObject(Object obj) { protectedMap.put(0, obj); } // Returns the same instance every time public static Object getObject() { return protectedMap.get(0); } // Unprotect the objects so that they can be garbage-collected public static void unpreserveObject() { protectedMap.remove(0); } }
This is a singleton class (see MSC07-J. Prevent multiple instantiations of singleton objects for more information on how to defensively code singleton classes). The initialization involves creating a background thread using the current instance of the class. The thread waits indefinitely by invoking Object.wait()
. Consequently, this object persists for the remainder of the Java Virtual Machine's (JVM) lifetime. Because the object is managed by a daemon thread, the thread cannot interfere with normal shutdown of the JVM.
Although the initialization involves a background thread, that thread neither accesses fields nor creates any liveness or safety issues. Consequently, this code is a safe and useful exception to this rule.
Risk Assessment
Starting and using background threads during class initialization can result in deadlock.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
TSM02-J | Low | Probable | High | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Parasoft Jtest | 2024.2 | CERT.TSM02.CSTART | Do not call the "start" method of threads from inside a constructor |
SonarQube | 9.9 | S2693 | Threads should not be started in constructors |
Bibliography
Chapter 8, "Lazy Initialization" | |
Chapter 5, "Creational Patterns, Singleton" |
12 Comments
David Svoboda
flag
variable? To indicate if the initialization succeeded?flag
variable necessary? That is, no thread will ever see the Lazy class loaded without a valid database connection (assuming it throws an exception if the connection fails). Prob should add the same 'set-flag-true-if-init-succeeds' that the 2nd CS has.Dhruv Mohindra
David Svoboda
OK, I changed the bool to an int in the first two code samples. The third sample is unchanged, as the flag has a clear purpose there.
Dhruv Mohindra
I think the third can also be changed. I think in the third case, it is better to initialize
number
in a static initializer or assign a value to it when it is declared.Dhruv Mohindra
David, can we keep the old title for the time being? Titles with "take care", "understand", "assume" are very hard to enforce...
David Svoboda
I wanted the
flag
to be distinct in the third code sample, b/c it indicates a successful database connection...theflag
vars in the first two samples didn't do anything, so they should have had different names and/or types. They do nowWe can change the title back, as long as we are truly acknowledging that there are safe exceptions to the rule. IOW this code works, but don't try this at home, kids.
Dhruv Mohindra
Static initialization is guaranteed to be atomic, right? Then why the flag?
David Svoboda
To indicate if the connection gets successfully created. I tweaked the code to make this more clear.
Dhruv Mohindra
EDIT: I've made the change described below and some others...
David, I think we could get rid of the flag and defer the check for null to the caller of getConnection(). If not, this method should check flag itself before returning the connection else throw an exception.
Robert Seacord (Manager)
resolved issue. "can" should be used in preference to "may"
I added "for example" which should be used and not "for instance".
James Ahlborn
I don't see the point of the protectedMap in the ObjectPreserver example for TSM02-EX0. you could simply use a volatile reference to the object to be preserved.
A Bishop
Automated Detection?