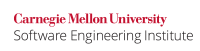
Many functions accept pointers as arguments. If the function dereferences an invalid pointer (as in EXP34-C. Do not dereference null pointers) or reads or writes to a pointer that does not refer to an object, the results are undefined. Typically, the program will terminate abnormally when an invalid pointer is dereferenced, but it is possible for an invalid pointer to be dereferenced and its memory changed without abnormal termination [Jack 2007]. Such programs can be difficult to debug because of the difficulty in determining if a pointer is valid.
One way to eliminate invalid pointers is to define a function that accepts a pointer argument and indicates whether or not the pointer is valid for some definition of valid. For example, the following function declares any pointer to be valid except NULL
:
int valid(void *ptr) { return (ptr != NULL); }
Some platforms have platform-specific pointer validation tools.
The following code relies on the _etext
address, defined by the loader as the first address following the program text on many platforms, including AIX, Linux, QNX, IRIX, and Solaris. It is not POSIX-compliant, nor is it available on Windows.
#include <stdio.h> #include <stdlib.h> int valid(void *p) { extern char _etext; return (p != NULL) && ((char*) p > &_etext); } int global; int main(void) { int local; printf("pointer to local var valid? %d\n", valid(&local)); printf("pointer to static var valid? %d\n", valid(&global)); printf("pointer to function valid? %d\n", valid((void *)main)); int *p = (int *) malloc(sizeof(int)); printf("pointer to heap valid? %d\n", valid(p)); printf("pointer to end of allocated heap valid? %d\n", valid(++p)); free(--p); printf("pointer to freed heap valid? %d\n", valid(p)); printf("null pointer valid? %d\n", valid(NULL)); return 0; }
On a Linux platform, this program produces the following output:
pointer to local var valid? 1 pointer to static var valid? 1 pointer to function valid? 0 pointer to heap valid? 1 pointer to end of allocated heap valid? 1 pointer to freed heap valid? 1 null pointer valid? 0
The valid()
function does not guarantee validity; it only identifies null pointers and pointers to functions as invalid. However, it can be used to catch a substantial number of problems that might otherwise go undetected.
Noncompliant Code Example
In this noncompliant code example, the incr()
function increments the value referenced by its argument. It also ensures that its argument is not a null pointer. But the pointer could still be invalid, causing the function to corrupt memory or terminate abnormally.
void incr(int *intptr) { if (intptr == NULL) { /* Handle error */ } *intptr++; }
Compliant Solution
This incr()
function can be improved by using the valid()
function. The resulting implementation is less likely to dereference an invalid pointer or write to memory that is outside the bounds of a valid object.
void incr(int *intptr) { if (!valid(intptr)) { /* Handle error */ } *intptr++; }
The valid()
function can be implementation dependent and perform additional, platform-dependent checks when possible. In the worst case, the valid()
function may only perform the same null-pointer check as the noncompliant code example. However, on platforms where additional pointer validation is possible, the use of a valid()
function can provide checks.
Risk Assessment
A pointer validation function can be used to detect and prevent operations from being performed on some invalid pointers.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MEM10-C | High | Unlikely | High | P3 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
LDRA tool suite | 9.7.1 | 159 S | Enhanced enforcement |
Polyspace Bug Finder | R2023b | Memory allocation with tainted size | Size argument to memory function is from an unsecure source Pointer returned from dynamic allocation not checked for Pointer from an unsecure source may be NULL or point to unknown memory |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
SEI CERT C++ Coding Standard | VOID MEM10-CPP. Define and use a pointer validation function |
MITRE CWE | CWE-20, Improper Input Validation |