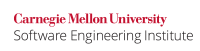
An object that has volatile-qualified type may be modified in ways unknown to the implementation or have other unknown side effects. Referencing a volatile object by using a non-volatile lvalue is undefined behavior. The C Standard, 6.7.3 [ISO/IEC 9899:2011], states
If an attempt is made to refer to an object defined with a volatile-qualified type through use of an lvalue with non-volatile-qualified type, the behavior is undefined.
Noncompliant Code Example
In this noncompliant code example, a volatile object is accessed through a non-volatile-qualified reference, resulting in undefined behavior:
#include <stdio.h> void func(void) { static volatile int **ipp; static int *ip; static volatile int i = 0; printf("i = %d.\n", i); ipp = &ip; /* May produce a warning diagnostic */ ipp = (int**) &ip; /* Constraint violation; may produce a warning diagnostic */ *ipp = &i; /* Valid */ if (*ip != 0) { /* Valid */ /* ... */ } }
The assignment ipp = &ip
is not safe because it allows the valid code that follows to reference the value of the volatile object i
through the non-volatile-qualified reference ip
. In this example, the compiler may optimize out the entire if
block because *ip != 0
must be false if the object to which ip
points is not volatile.
Implementation Details
This example compiles without warning on Microsoft Visual Studio 2013 when compiled in C mode (/TC
) but causes errors when compiled in C++ mode (/TP
).
GCC 4.8.1 generates a warning but compiles successfully.
Compliant Solution
In this compliant solution, ip
is declared volatile
:
#include <stdio.h> void func(void) { static volatile int **ipp; static volatile int *ip; static volatile int i = 0; printf("i = %d.\n", i); ipp = &ip; *ipp = &i; if (*ip != 0) { /* ... */ } }
Risk Assessment
Accessing an object with a volatile-qualified type through a reference with a non-volatile-qualified type is undefined behavior.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP32-C | Low | Likely | Medium | P6 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Clang | 3.9 | -Wincompatible-pointer-types-discards-qualifiers | |
Compass/ROSE |
|
|
|
GCC | 4.3.5 |
| Can detect violations of this rule when the |
LDRA tool suite | 9.7.1 | 344 S | Partially implemented |
Parasoft C/C++test | 9.5 | MISRA2004-11_5 | Fully implemented |
Polyspace Bug Finder | R2016a | Qualifier removed in conversion | Do not access a volatile object through a nonvolatile reference |
PRQA QA-C | Unable to render {include} The included page could not be found. | 0312,563,674 | Fully implemented |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
ISO/IEC TR 24772:2013 | Pointer Casting and Pointer Type Changes [HFC] Type System [IHN] |
MISRA C:2012 | Rule 11.8 (required) |
SEI CERT C++ Coding Standard | EXP55-CPP. Do not access a cv-qualified object through a cv-unqualified type |
Bibliography
[ISO/IEC 9899:2011] | 6.7.3, "Type Qualifiers" |