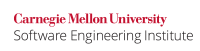
The assert()
statement is a convenient mechanism for incorporating diagnostic tests in code. Expressions used with the standard assert
statement should not have side effects. Typically, the behavior of the assert
statement depends on the status of a runtime property. If enabled, the assert
statement is designed to evaluate its expression argument and throw an AssertionError
if the result of the expression is convertible to false
. If disabled, assert
is defined to be a no-operation. Consequently, any side effects resulting from evaluation of the expression in the assertion are lost in production quality code.
Noncompliant Code Example
This noncompliant code example demonstrates an action being carried out in an assertion. The idea is to delete all the null
names from the list, however, the boolean
expression is unexpectedly not evaluated.
void process(int index) { assert names.remove(null); /* side effect */ /* ... */ }
Compliant Solution
Avoid the possibility of side effects in assertions. This can be achieved by decoupling the boolean
expression from the assertion.
void process(int index) { boolean nullsRemoved = names.remove(null); assert nullsRemoved; /* no side effect */ /* ... */ }
Risk Assessment
Side effects in assertions can lead to unexpected and erroneous behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP31- J |
low |
unlikely |
low |
P3 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C Coding Standard as EXP31-C. Avoid side effects in assertions.
This rule appears in the C++ Coding Standard as EXP31-CPP. Avoid side effects in assertions.
References
[Tutorials 08] Programming With Assertions
EXP30-J. Do not depend on operator precedence while using expressions containing side-effects 03. Expressions (EXP) EXP32-J. Do not use the equal and not equal operators to compare boxed primitives