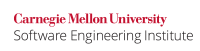
For a non-final class, if a constructor throws an exception before fully initializing the object, it becomes possible to maliciously obtain its instance. For example, an attack that uses the finalizer
construct allows an attacker to invoke arbitrary methods within the class despite of all authorization measures.
Noncompliant Code Example
The constructor of BankOperations
class performs the SSN validation using performSSNVerification()
. Assume that an attacker does not know the correct SSN, as a result this method trivially returns false
in this example. A SecurityException
is forcefully thrown as a result. The UserApp
class appropriately catches this and an access denied message is displayed. However, it is still possible for a malicious program to invoke methods of the partially initialized class BankOperations
. This is illustrated in the code that follows this example.
public class BankOperations { public BankOperations() { if (!performSSNVerification()) { throw new SecurityException("Invalid SSN!"); } } private boolean performSSNVerification() { return false; //returns true if data entered is valid, else false. Assume that the attacker just enters invalid SSN. } public static void greet() { System.out.println("Welcome user! You may now use all the features."); } } public class UserApp { public static void main(String[] args) { BankOperations bo; try { bo = new BankOperations(); } catch(SecurityException ex) { bo = null; } if(bo != null) bo.greet(); else System.out.println("Sorry, you were denied access to the features."); } }
To exploit this code, an attacker extends the BankOperations
class and overrides the finalizer
method. The gist of the attack is the capture of a handle of the partially initialized class. When the constructor throws an exception, the garbage collector waits to grab the object reference. However, by overriding the finalizer
, a reference is obtained using the this
keyword. Consequently, any method on the base class can be invoked maliciously. Note that, even a security manager check can be bypassed this way.
public class Interceptor extends BankOperations { private static Interceptor stealInstance = null; public static Interceptor get() { try { new Interceptor(); } catch(Exception ex) { } // ignore the exception try { synchronized(Interceptor.class) { while (stealInstance == null) { System.gc(); Interceptor.class.wait(10); } } } catch(InterruptedException ex) { return null; } return stealInstance; } public void finalize() { synchronized(Interceptor.class) { stealInstance = this; Interceptor.class.notify(); } System.out.println("Stolen the instance in finalize of " + this); } } public class AttackerApp { //invoke this class and gain access to the restrictive features public static void main(String[] args) { Interceptor i = Interceptor.get(); i.greet(); //now invoke any method of BankOperations class UserApp.main(args); //Invoke the original UserApp } }
This code is an exception to OBJ02-J. Avoid using finalizers
Compliant Solution
One compliant solution is to use an initialization flag that is explicitly set after the constructor has finished initializing the class. This flag should be tested before code is executed in any method. This (rather) imposing solution is recommended for security sensitive applications. For others, it is reasonable to initialize the default values of class variables to something like null
. \Check this
public class BankOperations { public static boolean initialized = false; public BankOperations() { if (!performSSNVerification()) { throw new SecurityException("Invalid SSN!"); } else initialized = true; } private boolean performSSNVerification() { return false; } public static void greet() { if(initialized == true) { System.out.println("Welcome user! You may now use all the features."); //other authorized code } else System.out.println("You are not permitted!"); } }
Compliant Solution
Another compliant solution is to declare the partially-initialized class final.
public final class BankOperations { ...
Risk Assessment
Allowing a partially initialized object to be accessed can provide an attacker with an opportunity to exploit the object.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ32-J |
medium |
probable |
high |
P?? |
L?? |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[SCG 07]]
[[Kabutz 01]] Issue 032 - Exceptional Constructors - Resurrecting the dead
[[JLS 05]] Section 12.6, Finalization of Class Instances
[[Bloch 08]] Item 7, Avoid finalizers
[[Darwin 04]] Section 9.5, The Finalize Method
[[Flanagan 05]] Section 3.3, Destroying and Finalizing Objects
[[API 06]] finalize()