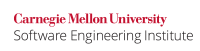
The assert()
statement is a convenient mechanism for incorporating diagnostic tests in code. Expressions used with the standard assert
statement should avoid side-effects. Typically, the behavior of the assert
statement depends on the status of a runtime property. When enabled, the assert
statement is designed to evaluate its expression argument and throw an AssertionError
if the result of the expression is false
. When disabled, assert
is defined to be a no-operation. Consequently, any side-effects resulting from evaluation of the expression in the assertion are lost when assertions are disabled.
Noncompliant Code Example
This noncompliant code example demonstrates an action being carried out in an assertion. The idea is to delete all the null
names from the list; however, the boolean
expression is unexpectedly not evaluated when assertions are disabled.
void process(int index) { assert names.remove(null); // side-effect // ... }
Compliant Solution
Avoid the possibility of side-effects in assertions. This can be achieved by decoupling the boolean
expression from the assertion.
void process(int index) { boolean nullsRemoved = names.remove(null); assert nullsRemoved; // no side-effect // ... }
Risk Assessment
Side-effects in assertions results in program behavior that depends on whether assertions are enabled or disabled.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP10-J |
low |
unlikely |
low |
P3 |
L3 |
Automated Detection
Automated detection of assertion operands that contain locally-visible side-effects is straightforward. Some analyses could require programmer assistance to determine which method invocations could contain side-effects.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
C Coding Standard: EXP31-C. Avoid side effects in assertions
C++ Coding Standard: EXP31-CPP. Avoid side effects in assertions
Bibliography
[Tutorials 2008] Programming With Assertions
EXP09-J. Do not allow an expression to write more than once to the same variable Expressions (EXP) EXP11-J. Ensure that autoboxed values have the intended type